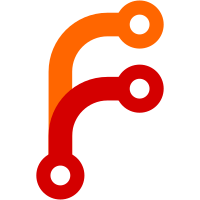
config: Felder catnamelong+cataccno neu + tests, line: feld category_view neu für spaltenansicht in kassenbuch + tests,
156 lines
5 KiB
Python
156 lines
5 KiB
Python
# -*- coding: utf-8 -*-
|
|
# This file is part of the cashbook-module from m-ds for Tryton.
|
|
# The COPYRIGHT file at the top level of this repository contains the
|
|
# full copyright notices and license terms.
|
|
|
|
from trytond.tests.test_tryton import ModuleTestCase, with_transaction
|
|
from trytond.pool import Pool
|
|
from trytond.transaction import Transaction
|
|
from trytond.exceptions import UserError
|
|
from trytond.modules.company.tests import create_company
|
|
|
|
|
|
class CategoryTestCase(ModuleTestCase):
|
|
'Test cashbook categoy module'
|
|
module = 'cashbook'
|
|
|
|
def prep_category(self, name='Cat1'):
|
|
""" create category
|
|
"""
|
|
pool = Pool()
|
|
Company = pool.get('company.company')
|
|
Category = pool.get('cashbook.category')
|
|
|
|
company = Company.search([])
|
|
if len(company) > 0:
|
|
company = company[0]
|
|
else :
|
|
company = create_company(name='m-ds')
|
|
|
|
category, = Category.create([{
|
|
'company': company.id,
|
|
'name': name,
|
|
}])
|
|
return category
|
|
|
|
@with_transaction()
|
|
def test_category_create_nodupl_at_root(self):
|
|
""" create category, duplicates are allowed at root-level
|
|
"""
|
|
pool = Pool()
|
|
Category = pool.get('cashbook.category')
|
|
|
|
company = create_company(name='m-ds')
|
|
|
|
with Transaction().set_context({
|
|
'company': company.id,
|
|
}):
|
|
cat1, = Category.create([{
|
|
'name': 'Test 1',
|
|
'description': 'Info',
|
|
}])
|
|
self.assertEqual(cat1.name, 'Test 1')
|
|
self.assertEqual(cat1.rec_name, 'Test 1')
|
|
self.assertEqual(cat1.description, 'Info')
|
|
self.assertEqual(cat1.company.rec_name, 'm-ds')
|
|
self.assertEqual(cat1.parent, None)
|
|
|
|
# duplicate, allowed
|
|
cat2, = Category.create([{
|
|
'name': 'Test 1',
|
|
'description': 'Info',
|
|
}])
|
|
self.assertEqual(cat2.name, 'Test 1')
|
|
self.assertEqual(cat2.rec_name, 'Test 1')
|
|
self.assertEqual(cat2.description, 'Info')
|
|
self.assertEqual(cat2.company.rec_name, 'm-ds')
|
|
self.assertEqual(cat2.parent, None)
|
|
|
|
@with_transaction()
|
|
def test_category_create_nodupl_diff_level(self):
|
|
""" create category
|
|
"""
|
|
pool = Pool()
|
|
Category = pool.get('cashbook.category')
|
|
|
|
company = create_company(name='m-ds')
|
|
|
|
with Transaction().set_context({
|
|
'company': company.id,
|
|
}):
|
|
cat1, = Category.create([{
|
|
'name': 'Test 1',
|
|
'description': 'Info',
|
|
'childs': [('create', [{
|
|
'name': 'Test 1',
|
|
}])],
|
|
}])
|
|
self.assertEqual(cat1.name, 'Test 1')
|
|
self.assertEqual(cat1.rec_name, 'Test 1')
|
|
self.assertEqual(cat1.description, 'Info')
|
|
self.assertEqual(cat1.company.rec_name, 'm-ds')
|
|
|
|
self.assertEqual(len(cat1.childs), 1)
|
|
self.assertEqual(cat1.childs[0].rec_name, 'Test 1/Test 1')
|
|
|
|
@with_transaction()
|
|
def test_category_create_deny_dupl_at_sublevel(self):
|
|
""" create category
|
|
"""
|
|
pool = Pool()
|
|
Category = pool.get('cashbook.category')
|
|
|
|
company = create_company(name='m-ds')
|
|
|
|
with Transaction().set_context({
|
|
'company': company.id,
|
|
}):
|
|
self.assertRaisesRegex(UserError,
|
|
'The category name already exists at this level.',
|
|
Category.create,
|
|
[{
|
|
'name': 'Test 1',
|
|
'description': 'Info',
|
|
'childs': [('create', [{
|
|
'name': 'Test 1',
|
|
}, {
|
|
'name': 'Test 1',
|
|
}])],
|
|
}])
|
|
|
|
@with_transaction()
|
|
def test_category_create_with_account(self):
|
|
""" create category + account
|
|
"""
|
|
pool = Pool()
|
|
Account = pool.get('account.account')
|
|
Category = pool.get('cashbook.category')
|
|
|
|
company = create_company(name='m-ds')
|
|
|
|
with Transaction().set_context({
|
|
'company': company.id,
|
|
}):
|
|
account, = Account.create([{
|
|
'name': 'Account No 1',
|
|
'code': '0123',
|
|
}])
|
|
|
|
cat1, = Category.create([{
|
|
'name': 'Test 1',
|
|
'description': 'Info',
|
|
'account': account.id,
|
|
}])
|
|
self.assertEqual(cat1.name, 'Test 1')
|
|
self.assertEqual(cat1.rec_name, 'Test 1 [0123]')
|
|
self.assertEqual(cat1.description, 'Info')
|
|
self.assertEqual(cat1.company.rec_name, 'm-ds')
|
|
|
|
self.assertEqual(Category.search_count([
|
|
('account_code', '=', '0123'),
|
|
]), 1)
|
|
self.assertEqual(Category.search_count([
|
|
('account_code', '=', '123'),
|
|
]), 0)
|
|
|
|
# end CategoryTestCase
|