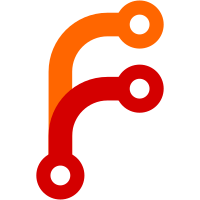
They are no more needed in Python3 and usually result in unexpected
behavior when wrongly used (like e.g. the one introduced in
764cacc091
and solved meanwhile by
refactorization).
97 lines
3.1 KiB
Python
97 lines
3.1 KiB
Python
# -*- coding: utf-8 -*-
|
|
# This file is part of the edocument-module for Tryton from m-ds.de.
|
|
# The COPYRIGHT file at the top level of this repository contains the
|
|
# full copyright notices and license terms.
|
|
|
|
import genshi.template
|
|
import os
|
|
from decimal import Decimal
|
|
from trytond.modules.edocument_uncefact.edocument import Invoice
|
|
from .mixin import EdocumentMixin
|
|
|
|
|
|
class XRechnung(EdocumentMixin, Invoice):
|
|
'EDocument XRechnung'
|
|
__name__ = 'edocument.xrechnung.invoice'
|
|
|
|
def sales_order_nums(self):
|
|
""" get string of sale-numbers
|
|
"""
|
|
if getattr(self.invoice, 'sales', None) is not None:
|
|
return ', '.join([x.number for x in self.invoice.sales])
|
|
|
|
def negate_amount(self, amount):
|
|
""" amount * -1.0
|
|
"""
|
|
if amount is not None and amount:
|
|
if isinstance(amount, Decimal):
|
|
return amount.copy_negate()
|
|
elif isinstance(amount, float):
|
|
return -1.0 * amount
|
|
elif isinstance(amount, int):
|
|
return -1 * amount
|
|
else:
|
|
return amount
|
|
|
|
def prepaid_amount(self, invoice):
|
|
""" compute already paid amount
|
|
"""
|
|
return invoice.total_amount - invoice.amount_to_pay
|
|
|
|
def invoice_note(self):
|
|
""" get 'description' + 'comment'
|
|
"""
|
|
notes = []
|
|
if self.invoice.description:
|
|
notes.append(self.invoice.description)
|
|
|
|
if self.invoice.comment:
|
|
notes.extend(self.invoice.comment.split('\n'))
|
|
if notes:
|
|
return '; '.join(notes)
|
|
|
|
def _get_template(self, version):
|
|
""" load our own template if 'version' is ours
|
|
"""
|
|
loader = genshi.template.TemplateLoader(
|
|
os.path.join(os.path.dirname(__file__), 'template'),
|
|
auto_reload=True)
|
|
|
|
if version in ['XRechnung-2.2', 'XRechnung-2.3', 'XRechnung-3.0']:
|
|
file_name = {
|
|
'380': 'XRechnung_invoice.xml',
|
|
'389': 'XRechnung_invoice.xml',
|
|
'381': 'XRechnung_credit.xml',
|
|
'261': 'XRechnung_credit.xml',
|
|
}.get(self.type_code)
|
|
|
|
if file_name:
|
|
return loader.load(os.path.join(version, file_name))
|
|
else:
|
|
raise ValueError('invalid type-code "%s"' % self.type_code)
|
|
else:
|
|
return super()._get_template(version)
|
|
|
|
# end XRechnung
|
|
|
|
|
|
class FacturX(EdocumentMixin, Invoice):
|
|
'Factur-X'
|
|
__name__ = 'edocument.facturxext.invoice'
|
|
|
|
def _get_template(self, version):
|
|
""" load our own template if 'version' is ours
|
|
"""
|
|
loader = genshi.template.TemplateLoader(
|
|
os.path.join(os.path.dirname(__file__), 'template'),
|
|
auto_reload=True)
|
|
|
|
if version == 'Factur-X-1.07.2-extended':
|
|
if self.type_code in ['380', '389', '381', '261']:
|
|
return loader.load(os.path.join(version, 'invoice.xml'))
|
|
else:
|
|
raise ValueError('invalid type-code "%s"' % self.type_code)
|
|
else:
|
|
return super()._get_template(version)
|
|
|
|
# end FacturX
|